I am a big fan of Chrome’s webrtc-internals tool. It is one of the most useful debugging tools for WebRTC and when it was added to Chrome back in 2012 it made my life a lot easier. I even wrote a lengthy series of blog post together with Tsahi Levent-Levi describing how to use it to debug issues recently.
Firefox has a similar about:webrtc page which shows the local and remote SDP for each page as well as a very useful grid of ICE candidates. But unlike Chrome it does not show the exact order of API calls or nice graphs obtained from the getStats API. I miss both features dearly. Edge and Safari don’t support similar debugging helpers currently either.
It is hard to support a WebRTC service using several distinct tools with different capabilities. Wouldn’t it be nice if there was a universal WebRTC debug tool with everything I needed? Of course, so I decided to make one. I ended up taking some old code from our WebRTC Notifier and porting it to a WebExtension. The result is pretty amazing:
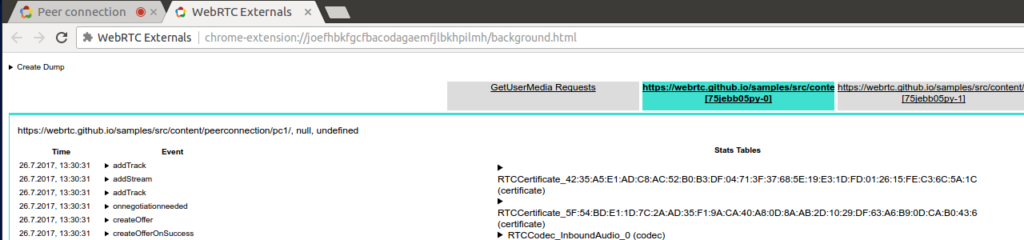
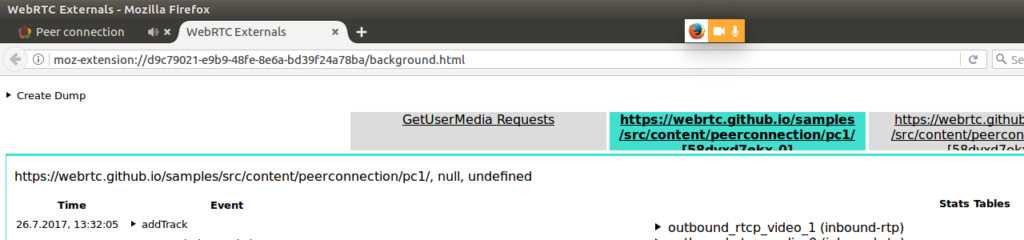
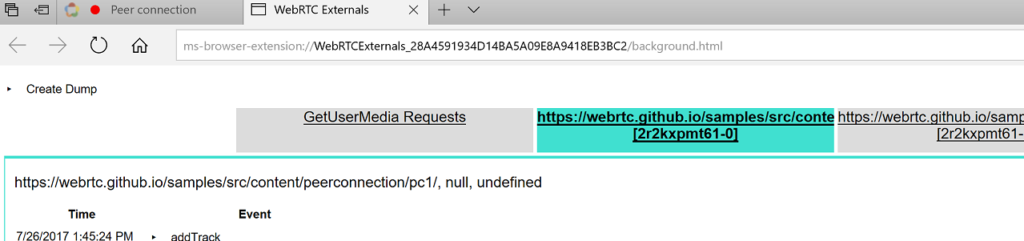
And I get a single tool workflow for debugging WebRTC issues that works in three different browsers. Hopefully Safari support will come soon too – the technique of intercepting the API calls is already working in Safari, so we just need to wait for support of the WebExtension APIs.
How to install the extension?
As this is a developer tool in the very early stages it has not been published to many extension stores. If there is enough interest it might make it to the extension stores, but for now you’ll have to manually install it using the instructions found here.
First clone the repository from github:
git clone https://github.com/fippo/webrtc-externals
Then follow these steps for each browser you want to setup:
Chrome
- Go to chrome://extensions/
- Enable developer via the checkbox in the upper right
- Click load unpacked extension
- Select the directory where you cloned the extension
- The WebRTC logo should appear in the top right corner and click on it
- Load your WebRTC page
Alternatively, check the official instructions here.
Firefox
- Use the web ext tool to run the extension
- Open a terminal
- Type this:
123npm install --global web-extcd webrtc-externals #Go to the directory where you cloned webrtc-externalsweb-ext run
- The WebRTC logo should appear in the top right corner and click on it
- Load your WebRTC page
Soon the addon will be installable directly from addons.mozilla.org.
Microsoft Edge
- Follow the steps from this description to load the extension in Microsoft Edge:
- Load about:flags in Edge
- Click on “Enable extension developer features (this might put your device at risk) “
- Go to the menu bar -> More -> Extension -> Load extension
- Find the folder where you cloned the webrtc-externals repo & select the folder
- Check that the WebRTC logo appears in the top right corner and click on it
- Load your WebRTC page
How to use the extension?
Unfortunately using the extension is almost as complicated as webrtc-internals has become recently. Before opening the page with WebRTC that you want to debug, first make sure that the background page is open by clicking on the WebRTC logo in the top right corner of your browser.
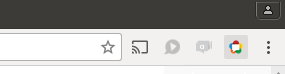
Clicking this button will open the background page in a new tab.
Next, go to the page you want to debug, for example one of the good old webrtc sample pages and make a call. It should look quite similar to what you expect from the Chrome webrtc-internals page.
How does it work?
WebExtensions are a (relatively) new approach to modifying browser capabilities. They basically take Chrome’s extension API and implement it in other browsers like Firefox and Edge. It started in 2015 and is now pretty mature so that you can easily write code that runs in all three browsers.
The extension consists of three pieces:
-
- a browser action which opens a background page
- A background page similar to chrome://webrtc-internals
- A content script which is injected into the page and intercepts all the peerConnection API calls
Then you need the webrtc-internals page and some glue to make the messaging between the different parts happen. Most of what we wrote about the webrtc-notify extension still applies to the general design.
Browser Action
You can see in the manifest that this loads a logo, the background page, and the content scripts described below.
Background page
For the background page it actually turned out to be very pragmatic to just copy both the HTML and Javascript over from Chrome’s webrtc-internals page. While that generates some console errors about chrome not being defined, it still works.
Content Script
The content script that intercepts the API calls is essentially an updated version of the old webrtc-notify extension mixed with some of the experiences Gustavo Garcia and me made while writing rtcstats, a JavaScript library that we use to log all peerConnection API calls to a server instead of a background page.
Once a webpage has finished loading the content script is executed. This ensures that the same RTCPeerConnection API is used even in browsers like Microsoft Edge where I prefer my own shim over the native implementation. The content script then overrides methods like createOffer on the RTCPeerConnection prototype, serializes the arguments and then calls the native method. It will also intercept the result of the native method for methods that have a callback like createOffer.
In addition to that there is a number of event listener for events like the icecandidate , addstream or track .
The content script will also poll getStats every second and send the data to the background page where they get visualized as you’re used to from webrtc-internals:
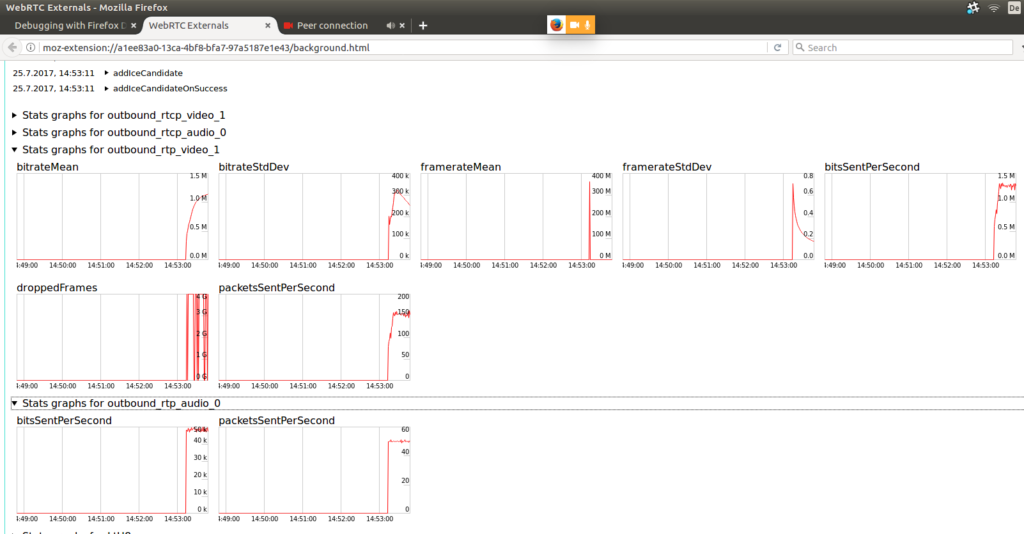
While I would strongly prefer to use the much nicer highcharts.js graphs reusing the existing code was much faster.
Caveats
Another thing to note is that currently the extension only works on sites that use the unprefixed RTCPeerConnection API. Notably Google Hangouts and even the fancy new Google Meet still relying on the old webkitRTCPeerConnection and navigator.webkitGetUserMedia APIs.
In the end…
Porting the old code to a WebExtension and getting it to run in three browsers was really easy. And getting a single workflow for debugging issues to work across browser is incredibly helpful for developing WebRTC applications. So this was a nice little summer hack but now it is time to get back to the beach!
{“author”: “Philipp Hancke“}
This is amazing, thanks for sharing!
heh, the topic surfaced again recently when you asked… 😉
Great work!!
It will help both WebRTC developers and users 😉
Does this work on the latest Edge? I’m seeing this on the background page console:
CONSOLE21301: serviceWorker.getRegistrations is rejected due to unsecure context or host restriction in ms-browser-extension://WebRTCExternals_08ECF9BFD4E04D48ABFB87F7C1F09E76/_generated_background_page.html.
Hi, maybe you know how I can use chrome://webrtc-internals/ for mobile Chrome (Android)? Yes, I can open it in a separate tab on the same phone, and can even switch between the tow tabs: the one that runs my webRTC client and the one that collects the stats. But this means that I cannot follow this in real time, and the 6″ screen is too small… And I can go to chrome://inspect/#devices on the connected PC, but it does not help me see the WebRTC stats of the device 🙁
Not supported. The closest thing you might try is spinning up https://github.com/jitsi/rtcstats with a custom server that transfers the data to another client for realtime display.